Vue JS Sort Array by Alphabet: Sorting an array of objects in alphabetical order is a common task in Vue.js development. To achieve this, one can use the sort
method available in JavaScript. In this article, we will cover the steps to create the array of objects, implement the ascending or descending alphabetical sort, and display the sorted array in Vue.js
What is the process for sorting an array of objects in a Vue.js
Vue.js is a JavaScript framework framework that allows developers to create dynamic and responsive user interfaces. One useful feature of Vue.js is its ability to sort arrays or tables by name. This can be achieved using the built-in sorting method of JavaScript arrays.
To sort an array alphabetically, developers can use the sort() method with a custom comparison function that compares each element in the array based on its name. For tables, this can be achieved by binding the table data to a computed property that uses the sort() method to sort the data based on the selected column.
Overall, Vue.js makes it easy to sort arrays or tables by name, allowing developers to create user interfaces that are both functional and intuitive.
Vue Js Sort Array of Objects by Alphabetical Order
<div id="app">
<button @click="toggleSortOrder">{{ sortOrderButtonText }}</button>
<table>
<tr>
<th>S.no</th>
<th>Developer_Name</th>
</tr>
<tr v-for="(item, index) in sortedItems">
<td>{{ index + 1 }}</td>
<td>{{ item.name }}</td>
</tr>
</table>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
items: [
{ id: 1, name: 'Andrew' },
{ id: 2, name: 'CodeSmith' },
{ id: 3, name: 'Softwize' },
{ id: 4, name: 'DevHive' },
{ id: 5, name: 'Matthew Taylor' },
{ id: 6, name: 'ByteBuilders' },
{ id: 7, name: 'Jennifer Lee' },
{ id: 8, name: 'Robert Thompson' },
{ id: 9, name: 'Christopher Davis' },
{ id: 10, name: 'Laura Smith' },
{ id: 11, name: 'William Johnson' },
{ id: 12, name: 'Elizabeth Martinez' },
{ id: 13, name: 'David Lee' },
{ id: 14, name: 'Andrew' },
{ id: 15, name: 'AlgoAllies' },
],
sortOrder: "asc"
};
},
computed: {
sortedItems() {
let sortedArray = [...this.items];
sortedArray.sort((a, b) => {
let comparison = 0;
if (a.name < b.name) {
comparison = -1;
} else if (a.name > b.name) {
comparison = 1;
}
return comparison;
});
if (this.sortOrder === "desc") {
sortedArray.reverse();
}
return sortedArray;
},
sortOrderButtonText() {
return this.sortOrder === "asc" ? "Sort Descending" : "Sort Ascending";
}
},
methods: {
toggleSortOrder() {
this.sortOrder = this.sortOrder === "asc" ? "desc" : "asc";
}
}
});
</script>
Output of Vue Js Array Sort by Alphabet | Table Sort by Name
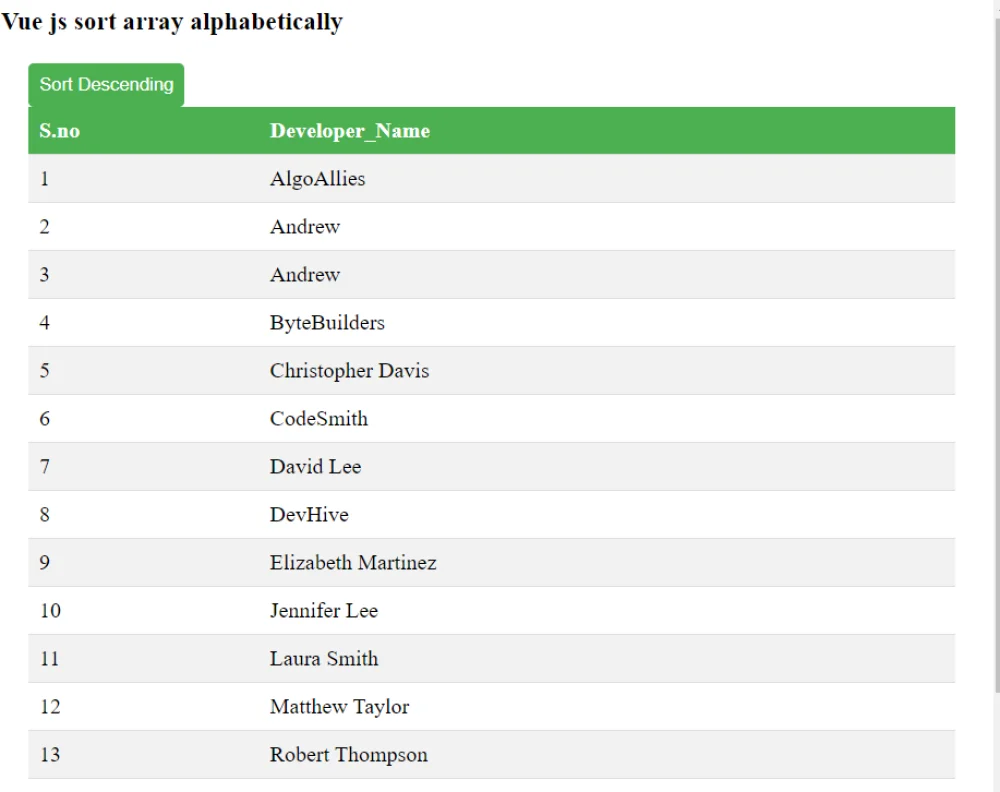